From cd8ceb283079e1e710ce2f9a77b218a4d1c81444 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Sun, 6 Nov 2022 19:40:41 +0100
Subject: [PATCH 01/17] Create create-mastermind-game-using-python.mdx
---
.../create-mastermind-game-using-python.mdx | 225 ++++++++++++++++++
1 file changed, 225 insertions(+)
create mode 100644 projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
diff --git a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
new file mode 100644
index 00000000..8f2db130
--- /dev/null
+++ b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
@@ -0,0 +1,225 @@
+---
+title: Create Mastermind game using Python
+author: 3t8
+datePublished: 2022-11-06
+description: Learn how to create Mastermind game using Python in this project tutorial
+header: URL
+tags:
+ - beginner
+ - python
+---
+
+# Create Mastermind game using Python
+
+
+
+
+
+**Prerequisites:** Python fundamentals
+**Versions:** Python 3.10
+**Read Time:** 30 minutes
+
+## [#](#-introduction) Introduction
+
+With more than 55 million units sold, [Mastermind](https://en.wikipedia.org/wiki/Mastermind_(board_game)) is a one of the world’s most popular strategy games ever. The idea behind it is to guess an unknown sequence of coloured pegs in the fewest number of attempts possible. Despite having simple rules the game is still very difficult and entertaining.
+
+Because representing colors in a terminal is not an easy task, we are going to create a more *modern* version of the Mastermind game. In 1977 Invicta released an electronic version of the game where the colored pegs were replaced by an array of up to five digits. In this tutorial we are going to recreate it in Python.
+
+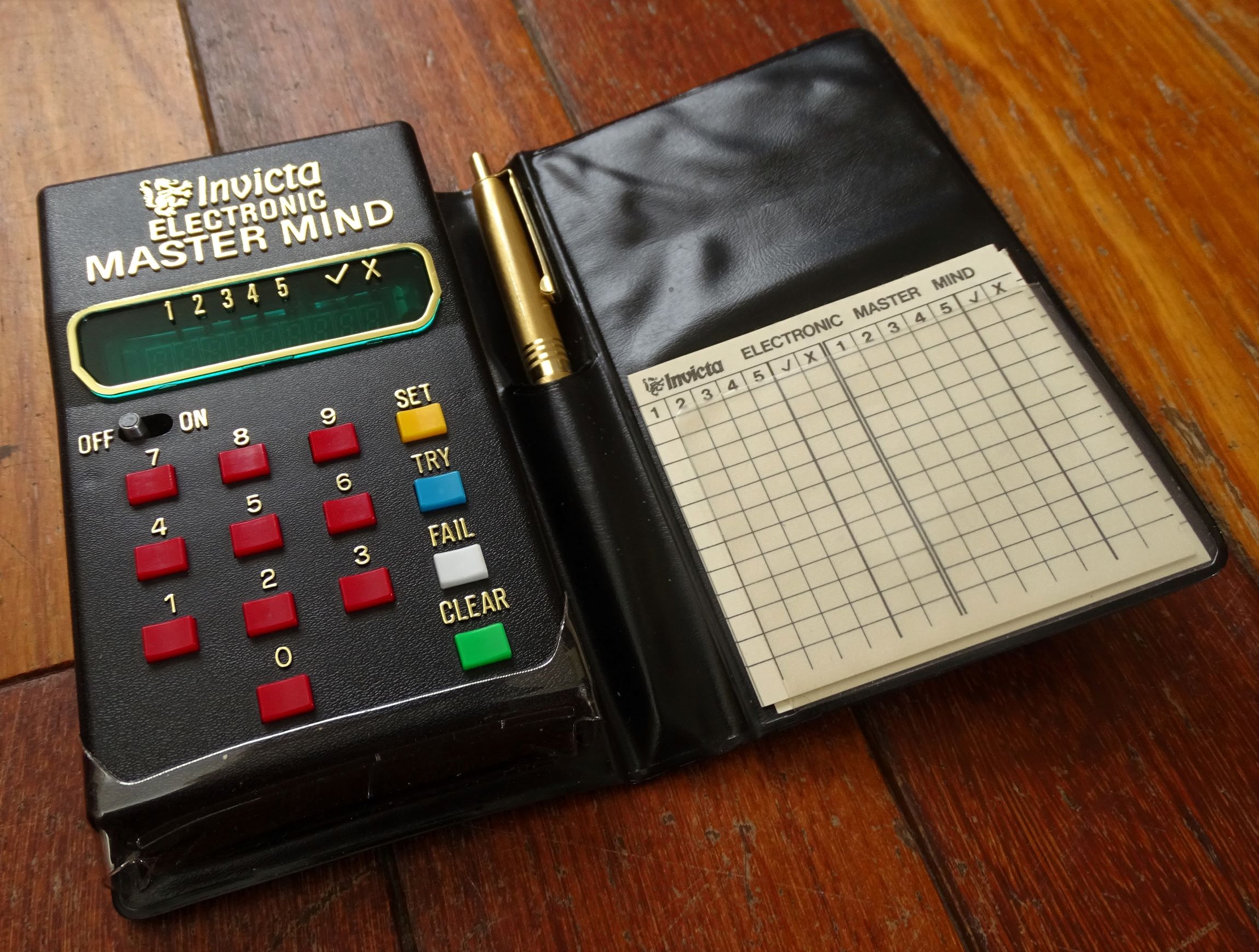
+
+## [#](#-rules) Rules
+
+The rules are really simple:
+- A random 4 digit secret code is generated
+- The player will input a 4 digit number trying to guess the secret code
+- The number of digits that are correct and in the right position is returned
+- The number of digits that are correct but in the wrong position is returned
+
+## [#](#-generate-a-random-number) Step 1. Generate a random number
+
+In order to generate a random number we need to use the `random` module. More specifically we are going to use `randrange` method.
+
+```python
+from random import randrange
+```
+
+The `randrange` method returns a random number within the specified range. To generate a 4 digit number we need our range to be between 0 and 10000 (the largest number generated would be 9999)
+
+```python
+number = randrange(10000)
+```
+
+There is only a small problem. If the generated number is smaller than 1000 we will end up with a secret code that has less than 4 digits.
+
+To fix that we need to add zeroes at the beginning of the number until it reaches 4 digits.
+
+The easiest way to do it is converting the number to a string and justify it to the right with `rjust` by adding `0` until it has `4` digits.
+
+``` python
+number = str(number).rjust(4, '0')
+```
+
+We now have our random secret code.
+
+## [#](#-read-users-guess) Step 2. Read user's guess
+
+The next step is getting user's guess. To do that we will use the `input` function.
+
+We use `isnumeric` to check that all the characters taken as input are numeric and `len(guess)` to make sure that the guess has 4 digits.
+
+If any of those conditions are not met, we will ask again the user for a guess.
+
+We set `guess = 0` to execute the instructions inside the while the first time.
+
+
+```python
+guess = 0
+while (guess.isnumeric() == False or len(guess) != 4):
+ guess = input('Guess the four digit number: ')
+```
+The `guess` read with `input` is treated as a string, same as the secret code `number`.
+
+## [#](#-additional-variables) Step 3. Additional variables
+
+We need a variable to count the correct digits in the right position
+
+```python
+correct_position = 0
+```
+
+and one to count the correct digits in the wrong position
+
+```python
+correct_digit = 0
+```
+We will also use a copy of `number` in order to keep track of the digits that have already been matched and the ones that are left unmatched
+
+```python
+unmatched = number
+```
+
+## [#](#-count-guessed-digits-in-the-right-position) Step 4. Count guessed digits in the right position
+
+To count how many digits of the `guess` match the ones in `number` we need to check all of them in pairs.
+Because there are 4 digits we need to iterate the check 4 times so we use `range(4)`.
+
+If both `guess` and `number` have the same digits in the same positions, we increment the `correct_position` counter.
+
+To keep track of the matched digits and exclude them later whene we count the right digits in the wrong positions, we conventionally set `unmatched` to `f` at the position of the matched digit. This way `f` will never match with any digit from 0 to 9
+
+```python
+for i in range(4):
+ if (guess[i] == number[i]):
+ unmatched = unmatched.replace(guess[i], 'f', 1)
+ correct_position += 1
+```
+
+## [#](#-count-guessed-digits-in-the-wrong-position) Step 5. Count guessed digits in the wrong position
+
+There is no need to count the right digits in the wrong position if we already matched all 4.
+
+```python
+if (correct_position < 4):
+```
+
+We will only execute the next instructions if the condition above is true.
+
+Once again we use `range(4)` to iterate over all 4 digits.
+This time the digits are compared between `guess` and `unmatched`.
+We check if `guess[i] in unmatched` and if that's true we increment the `correct_digit` counter.
+
+The `guess` variable has not been change at all but we **must not** check the digits that have already been matched in the right position.
+That is done by also checking if `guess[i] != number[i]` (or if `unmathced[i] != 'f'`).
+
+```python
+if (guess[i] in unmatched and guess[i] != number[i]):
+ unmatched = unmatched.replace(guess[i], 'f', 1)
+ correct_digit += 1
+```
+Like the last time, the matched digits in `unmatched` are set to `f` so they will be excluded in the next iteration.
+
+## [#](#-printing-guessed-digits-and-winning) Step 6. Printing guessed digits
+
+At this point both `correct_position` and `correct_digit` counters should have the right values. We just need to print them.
+
+```python
+print('Digits in right position: ' + str(correct_position))
+print('Digits in wrong position: ' + str(correct_digit))
+```
+
+## [#](#-counting-the-number-of-guesses-and-winning) Step 7. Counting the number of guesses and winning
+
+The whole code after the `number` generation needs to be inside a
+
+```python
+while (guess != number):
+```
+
+Each time the code is executed we need to increment the `tries` variable
+
+```python
+tries += 1
+```
+
+If the `while` condition is not satisfied that means `guess == number` so the secret number has been guessed.
+
+We tell the user that he won and we also tell him how many attempts it took
+
+```python
+print('You are a mastermind! You guessed the number in ' + str(tries) + ' tries')
+```
+
+## [#](#-code) Code
+
+The whole code should look like this:
+
+```python
+from random import randrange
+# Generate a random number from 0 to 10000
+number = randrange(10000)
+# Add padding to the number if it doesn't have four digits
+number = str(number).rjust(4, '0')
+tries = 0
+guess = 0
+
+while (guess != number):
+ tries += 1
+ guess = ''
+ # unmatched will keep track of the digits that have not been guessed
+ unmatched = number
+ correct_digit = 0
+ correct_position = 0
+ # Read a four digit number
+ while (guess.isnumeric() == False or len(guess) != 4):
+ guess = input('Guess the four digit number: ')
+ # Count the guessed digits in the right postion
+ for i in range(4):
+ if (guess[i] == number[i]):
+ # Set the guessed digit to 'f' in unmatched
+ unmatched = unmatched.replace(guess[i], 'f', 1)
+ correct_position += 1
+ # Count the guessed digits in the wrong position
+ if (correct_position < 4):
+ for i in range(4):
+ if (guess[i] in unmatched and guess[i] != number[i]):
+ # Set the guessed digit to 'f' in unmatched
+ unmatched = unmatched.replace(guess[i], 'f', 1)
+ correct_digit += 1
+ print('Digits in right position: ' + str(correct_position))
+ print('Digits in wrong position: ' + str(correct_digit))
+print('You are a mastermind! You guessed the number in ' + str(tries) + ' tries')
+```
+
+## [#](#-improvements) improvements
+
+To improve this project you could try to:
+
+- Increase the number of digits the user has to guess. With 4 digits the possible permutations are 10000.
+- Allow the user to use a [custom seed](https://docs.python.org/3/library/random.html#random.seed) for the secret number. This could allow multple users to compete against each other and try and guess the same number.
+- Try to code your own solver by implementing [Knuth's algorithm](https://en.wikipedia.org/wiki/Mastermind_(board_game)#Worst_case:_Five-guess_algorithm).
+
+## [#](#-more-resources) More Resources
+
+- [Solution on GitHub](https://github.com/codedex-io/projects/blob/main/projects/create-mastermind-game-using-python/mastermind.py)
+- [Documentation: random](https://docs.python.org/3/library/random.html)
+- [Mastermind solver](https://nebupookins.github.io/JS-Mastermind-Solver/)
From f26fc72cf9ef823effd9b7d4da9ac71da7e4db05 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Sun, 6 Nov 2022 19:41:51 +0100
Subject: [PATCH 02/17] Create mastermind.py
---
.../mastermind.py | 34 +++++++++++++++++++
1 file changed, 34 insertions(+)
create mode 100644 projects/create-mastermind-game-using-python/mastermind.py
diff --git a/projects/create-mastermind-game-using-python/mastermind.py b/projects/create-mastermind-game-using-python/mastermind.py
new file mode 100644
index 00000000..d7a47a0d
--- /dev/null
+++ b/projects/create-mastermind-game-using-python/mastermind.py
@@ -0,0 +1,34 @@
+from random import randrange
+# Generate a random number from 0 to 10000
+number = randrange(10000)
+# Add padding to the number if it doesn't have four digits
+number = str(number).rjust(4, '0')
+tries = 0
+guess = 0
+
+while (guess != number):
+ tries += 1
+ guess = ''
+ # unmatched will keep track of the digits that have not been guessed
+ unmatched = number
+ correct_digit = 0
+ correct_position = 0
+ # Read a four digit number
+ while (guess.isnumeric() == False or len(guess) != 4):
+ guess = input('Guess the four digit number: ')
+ # Count the guessed digits in the right postion
+ for i in range(4):
+ if (guess[i] == number[i]):
+ # Set the guessed digit to 'f' in unmatched
+ unmatched = unmatched.replace(guess[i], 'f', 1)
+ correct_position += 1
+ # Count the guessed digits in the wrong position
+ if (correct_position < 4):
+ for i in range(4):
+ if (guess[i] in unmatched and guess[i] != number[i]):
+ # Set the guessed digit to 'f' in unmatched
+ unmatched = unmatched.replace(guess[i], 'f', 1)
+ correct_digit += 1
+ print('Digits in right position: ' + str(correct_position))
+ print('Digits in wrong position: ' + str(correct_digit))
+print('You are a mastermind! You guessed the number in ' + str(tries) + ' tries')
From 80cf0b17c9a7513a19a05c597259f11d271ebcd3 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Wed, 23 Nov 2022 13:40:21 +0100
Subject: [PATCH 03/17] Update
projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
Co-authored-by: Sonny Li
---
.../create-mastermind-game-using-python.mdx | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
diff --git a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
index 8f2db130..60062e7a 100644
--- a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
+++ b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
@@ -1,5 +1,5 @@
---
-title: Create Mastermind game using Python
+title: Create Mastermind Game with Python
author: 3t8
datePublished: 2022-11-06
description: Learn how to create Mastermind game using Python in this project tutorial
From 6313cc542afabfef154d3f4f6557045dd76aafad Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Wed, 23 Nov 2022 13:44:49 +0100
Subject: [PATCH 04/17] Update
projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
Co-authored-by: Sonny Li
---
.../create-mastermind-game-using-python.mdx | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
diff --git a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
index 60062e7a..a8373715 100644
--- a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
+++ b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
@@ -9,7 +9,7 @@ tags:
- python
---
-# Create Mastermind game using Python
+# Create Mastermind Game with Python
From 199f29a0e49f0d0efcd129b559dee701ab7705e9 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Wed, 23 Nov 2022 13:44:58 +0100
Subject: [PATCH 05/17] Update
projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
Co-authored-by: Sonny Li
---
.../create-mastermind-game-using-python.mdx | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
diff --git a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
index a8373715..edc9dbf1 100644
--- a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
+++ b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
@@ -35,7 +35,7 @@ The rules are really simple:
- The number of digits that are correct and in the right position is returned
- The number of digits that are correct but in the wrong position is returned
-## [#](#-generate-a-random-number) Step 1. Generate a random number
+## [#](#-generate-a-random-number) Step 1. Generate a Random Number
In order to generate a random number we need to use the `random` module. More specifically we are going to use `randrange` method.
From 9eedf82fea45635b75d99c9e531d9b7aa4c1cda9 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Wed, 23 Nov 2022 13:45:10 +0100
Subject: [PATCH 06/17] Update
projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
Co-authored-by: Sonny Li
---
.../create-mastermind-game-using-python.mdx | 1 +
1 file changed, 1 insertion(+)
diff --git a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
index edc9dbf1..3f2468cc 100644
--- a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
+++ b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
@@ -175,6 +175,7 @@ The whole code should look like this:
```python
from random import randrange
+
# Generate a random number from 0 to 10000
number = randrange(10000)
# Add padding to the number if it doesn't have four digits
From 5b2d31903127f8f1ce7ad3cc5af16cccc034f554 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Wed, 23 Nov 2022 13:45:18 +0100
Subject: [PATCH 07/17] Update
projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
Co-authored-by: Sonny Li
---
.../create-mastermind-game-using-python.mdx | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
diff --git a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
index 3f2468cc..31bb6a57 100644
--- a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
+++ b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
@@ -211,7 +211,7 @@ while (guess != number):
print('You are a mastermind! You guessed the number in ' + str(tries) + ' tries')
```
-## [#](#-improvements) improvements
+## [#](#-improvements) Improvements
To improve this project you could try to:
From a8d8323255dd9cd17ec4f4f8765968e28485463f Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Wed, 23 Nov 2022 13:58:36 +0100
Subject: [PATCH 08/17] Fix typos
---
.../create-mastermind-game-using-python.mdx | 10 +++++-----
1 file changed, 5 insertions(+), 5 deletions(-)
diff --git a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
index 31bb6a57..df575bb7 100644
--- a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
+++ b/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
@@ -2,7 +2,7 @@
title: Create Mastermind Game with Python
author: 3t8
datePublished: 2022-11-06
-description: Learn how to create Mastermind game using Python in this project tutorial
+description: Learn how to create Mastermind game with Python in this project tutorial
header: URL
tags:
- beginner
@@ -21,7 +21,7 @@ tags:
## [#](#-introduction) Introduction
-With more than 55 million units sold, [Mastermind](https://en.wikipedia.org/wiki/Mastermind_(board_game)) is a one of the world’s most popular strategy games ever. The idea behind it is to guess an unknown sequence of coloured pegs in the fewest number of attempts possible. Despite having simple rules the game is still very difficult and entertaining.
+With more than 55 million units sold, [Mastermind](https://en.wikipedia.org/wiki/Mastermind_(board_game)) is a one of the world’s most popular strategy games ever. The idea behind it is to guess an unknown sequence of colored pegs in the fewest number of attempts possible. Despite having simple rules the game is still very difficult and entertaining.
Because representing colors in a terminal is not an easy task, we are going to create a more *modern* version of the Mastermind game. In 1977 Invicta released an electronic version of the game where the colored pegs were replaced by an array of up to five digits. In this tutorial we are going to recreate it in Python.
@@ -105,7 +105,7 @@ Because there are 4 digits we need to iterate the check 4 times so we use `range
If both `guess` and `number` have the same digits in the same positions, we increment the `correct_position` counter.
-To keep track of the matched digits and exclude them later whene we count the right digits in the wrong positions, we conventionally set `unmatched` to `f` at the position of the matched digit. This way `f` will never match with any digit from 0 to 9
+To keep track of the matched digits and exclude them later when we count the right digits in the wrong positions, we conventionally set `unmatched` to `f` at the position of the matched digit. This way `f` will never match with any digit from 0 to 9
```python
for i in range(4):
@@ -128,7 +128,7 @@ Once again we use `range(4)` to iterate over all 4 digits.
This time the digits are compared between `guess` and `unmatched`.
We check if `guess[i] in unmatched` and if that's true we increment the `correct_digit` counter.
-The `guess` variable has not been change at all but we **must not** check the digits that have already been matched in the right position.
+The `guess` variable has not been changed at all, but we **must not** check the digits that have already been matched in the right position.
That is done by also checking if `guess[i] != number[i]` (or if `unmathced[i] != 'f'`).
```python
@@ -216,7 +216,7 @@ print('You are a mastermind! You guessed the number in ' + str(tries) + ' tries'
To improve this project you could try to:
- Increase the number of digits the user has to guess. With 4 digits the possible permutations are 10000.
-- Allow the user to use a [custom seed](https://docs.python.org/3/library/random.html#random.seed) for the secret number. This could allow multple users to compete against each other and try and guess the same number.
+- Allow the user to use a [custom seed](https://docs.python.org/3/library/random.html#random.seed) for the secret number. This could allow multiple users to compete against each other and try and guess the same number.
- Try to code your own solver by implementing [Knuth's algorithm](https://en.wikipedia.org/wiki/Mastermind_(board_game)#Worst_case:_Five-guess_algorithm).
## [#](#-more-resources) More Resources
From d2fd59e8fc1b2d6ca4187409a966ddadd2a8aad7 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Wed, 23 Nov 2022 14:00:37 +0100
Subject: [PATCH 09/17] Rename project
---
...-using-python.mdx => create-mastermind-game-with-python.mdx} | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
rename projects/create-mastermind-game-using-python/{create-mastermind-game-using-python.mdx => create-mastermind-game-with-python.mdx} (99%)
diff --git a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx b/projects/create-mastermind-game-using-python/create-mastermind-game-with-python.mdx
similarity index 99%
rename from projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
rename to projects/create-mastermind-game-using-python/create-mastermind-game-with-python.mdx
index df575bb7..9f9760fc 100644
--- a/projects/create-mastermind-game-using-python/create-mastermind-game-using-python.mdx
+++ b/projects/create-mastermind-game-using-python/create-mastermind-game-with-python.mdx
@@ -221,6 +221,6 @@ To improve this project you could try to:
## [#](#-more-resources) More Resources
-- [Solution on GitHub](https://github.com/codedex-io/projects/blob/main/projects/create-mastermind-game-using-python/mastermind.py)
+- [Solution on GitHub](https://github.com/codedex-io/projects/blob/main/projects/create-mastermind-game-with-python/mastermind.py)
- [Documentation: random](https://docs.python.org/3/library/random.html)
- [Mastermind solver](https://nebupookins.github.io/JS-Mastermind-Solver/)
From a65b124ab1003e5ef6a9b5bcf926c783e6d82470 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Wed, 23 Nov 2022 14:01:49 +0100
Subject: [PATCH 10/17] Rename project folder
---
.../create-mastermind-game-with-python.mdx | 0
1 file changed, 0 insertions(+), 0 deletions(-)
rename projects/{create-mastermind-game-using-python => create-mastermind-game-with-python}/create-mastermind-game-with-python.mdx (100%)
diff --git a/projects/create-mastermind-game-using-python/create-mastermind-game-with-python.mdx b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
similarity index 100%
rename from projects/create-mastermind-game-using-python/create-mastermind-game-with-python.mdx
rename to projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
From c617b46a2af698a6aafcee478ec7cb555279386a Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Wed, 23 Nov 2022 14:02:23 +0100
Subject: [PATCH 11/17] Rename project folder
---
.../mastermind.py | 0
1 file changed, 0 insertions(+), 0 deletions(-)
rename projects/{create-mastermind-game-using-python => create-mastermind-game-with-python}/mastermind.py (100%)
diff --git a/projects/create-mastermind-game-using-python/mastermind.py b/projects/create-mastermind-game-with-python/mastermind.py
similarity index 100%
rename from projects/create-mastermind-game-using-python/mastermind.py
rename to projects/create-mastermind-game-with-python/mastermind.py
From 6bc015e3e657935b887997cf371250cf39b44e13 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Wed, 23 Nov 2022 14:10:52 +0100
Subject: [PATCH 12/17] Update formatting
---
.../create-mastermind-game-with-python.mdx | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
diff --git a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
index 9f9760fc..d1530c14 100644
--- a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
+++ b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
@@ -163,7 +163,7 @@ tries += 1
If the `while` condition is not satisfied that means `guess == number` so the secret number has been guessed.
-We tell the user that he won and we also tell him how many attempts it took
+We tell the user that he/she won and we also tell him/her how many attempts it took
```python
print('You are a mastermind! You guessed the number in ' + str(tries) + ' tries')
From 8c09b12144e4e3136b64ca30cd9cb9a80b0951f1 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Fri, 2 Dec 2022 12:58:12 +0100
Subject: [PATCH 13/17] Update
projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
Co-authored-by: Jerry Zhu <54565411+Bobliuuu@users.noreply.github.com>
---
.../create-mastermind-game-with-python.mdx | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
diff --git a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
index d1530c14..4a3c696c 100644
--- a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
+++ b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
@@ -21,7 +21,7 @@ tags:
## [#](#-introduction) Introduction
-With more than 55 million units sold, [Mastermind](https://en.wikipedia.org/wiki/Mastermind_(board_game)) is a one of the world’s most popular strategy games ever. The idea behind it is to guess an unknown sequence of colored pegs in the fewest number of attempts possible. Despite having simple rules the game is still very difficult and entertaining.
+With more than 55 million units sold, [Mastermind](https://en.wikipedia.org/wiki/Mastermind_(board_game)) is a one of the world’s most popular strategy games. The idea behind it is to guess an unknown sequence of colored pegs in the fewest number of attempts possible. Despite having simple rules, the game is still very difficult and entertaining.
Because representing colors in a terminal is not an easy task, we are going to create a more *modern* version of the Mastermind game. In 1977 Invicta released an electronic version of the game where the colored pegs were replaced by an array of up to five digits. In this tutorial we are going to recreate it in Python.
From a5fdb942571ee0e35845b98bf44b598d25b0b6af Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Fri, 2 Dec 2022 12:58:44 +0100
Subject: [PATCH 14/17] Update
projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
Co-authored-by: Jerry Zhu <54565411+Bobliuuu@users.noreply.github.com>
---
.../create-mastermind-game-with-python.mdx | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
diff --git a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
index 4a3c696c..ac9da0dd 100644
--- a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
+++ b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
@@ -23,7 +23,7 @@ tags:
With more than 55 million units sold, [Mastermind](https://en.wikipedia.org/wiki/Mastermind_(board_game)) is a one of the world’s most popular strategy games. The idea behind it is to guess an unknown sequence of colored pegs in the fewest number of attempts possible. Despite having simple rules, the game is still very difficult and entertaining.
-Because representing colors in a terminal is not an easy task, we are going to create a more *modern* version of the Mastermind game. In 1977 Invicta released an electronic version of the game where the colored pegs were replaced by an array of up to five digits. In this tutorial we are going to recreate it in Python.
+Since representing colors in a terminal is not an easy task, we are going to create a more *modern* version of the Mastermind game. In 1977 Invicta released an electronic version of the game where the colored pegs were replaced by an array of up to five digits. In this tutorial, we are going to recreate that version in Python.
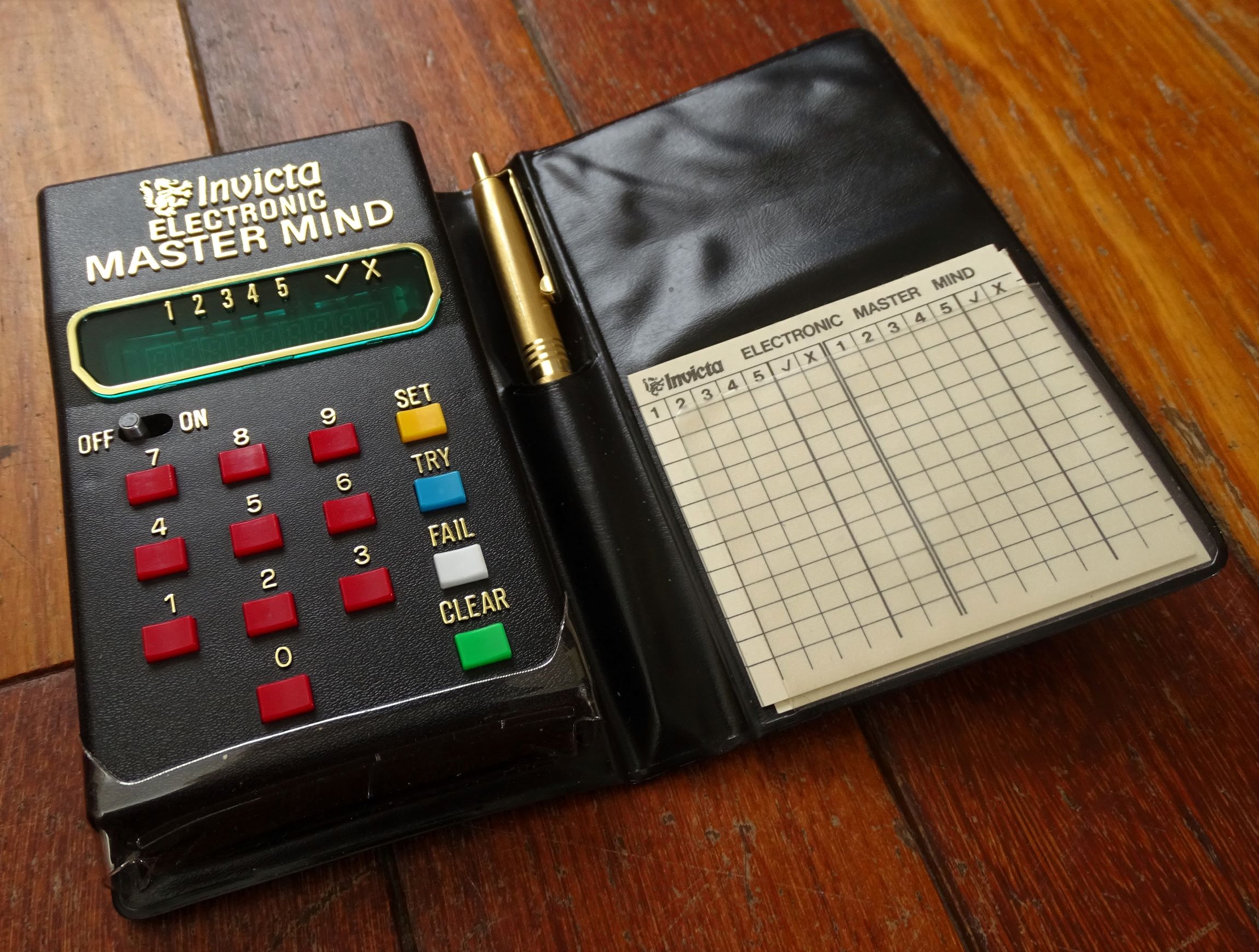
From cf405c452d1a6279b81aa426a3bddeb42e2beb44 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Fri, 2 Dec 2022 13:01:43 +0100
Subject: [PATCH 15/17] Update
projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
Co-authored-by: Jerry Zhu <54565411+Bobliuuu@users.noreply.github.com>
---
.../create-mastermind-game-with-python.mdx | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
diff --git a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
index ac9da0dd..593b0d90 100644
--- a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
+++ b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
@@ -31,7 +31,7 @@ Since representing colors in a terminal is not an easy task, we are going to cre
The rules are really simple:
- A random 4 digit secret code is generated
-- The player will input a 4 digit number trying to guess the secret code
+- The player will input a 4 digit number to try to guess the secret code
- The number of digits that are correct and in the right position is returned
- The number of digits that are correct but in the wrong position is returned
From 5090ba28b85a2f6e1e41af1e0bc80fc2fe144b54 Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Fri, 2 Dec 2022 13:01:56 +0100
Subject: [PATCH 16/17] Update
projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
Co-authored-by: Jerry Zhu <54565411+Bobliuuu@users.noreply.github.com>
---
.../create-mastermind-game-with-python.mdx | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
diff --git a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
index 593b0d90..7ce16f5a 100644
--- a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
+++ b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
@@ -63,7 +63,7 @@ We now have our random secret code.
## [#](#-read-users-guess) Step 2. Read user's guess
-The next step is getting user's guess. To do that we will use the `input` function.
+The next step is getting the user's guess. To do that, we will use the `input` function.
We use `isnumeric` to check that all the characters taken as input are numeric and `len(guess)` to make sure that the guess has 4 digits.
From 8d2d980d5543ba6ec47e2df14d5e00ed7cf09b1b Mon Sep 17 00:00:00 2001
From: 3t8 <62209650+3t8@users.noreply.github.com>
Date: Fri, 2 Dec 2022 13:05:30 +0100
Subject: [PATCH 17/17] Update
projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
Co-authored-by: Jerry Zhu <54565411+Bobliuuu@users.noreply.github.com>
---
.../create-mastermind-game-with-python.mdx | 2 +-
1 file changed, 1 insertion(+), 1 deletion(-)
diff --git a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
index 7ce16f5a..88328687 100644
--- a/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
+++ b/projects/create-mastermind-game-with-python/create-mastermind-game-with-python.mdx
@@ -67,7 +67,7 @@ The next step is getting the user's guess. To do that, we will use the `input` f
We use `isnumeric` to check that all the characters taken as input are numeric and `len(guess)` to make sure that the guess has 4 digits.
-If any of those conditions are not met, we will ask again the user for a guess.
+If any of those conditions are not met, we will ask the user for a guess again.
We set `guess = 0` to execute the instructions inside the while the first time.