getCustomer(String username) {
+ return Optional.ofNullable(customersByUsername.get(username));
+ }
+
+ /**
+ * Este método retorna um consumidor de maneira randômica.
+ *
+ * @param random Dado randômico / aleatório
+ * @return customersById Consumidor do Id escolhido
+ */
+ private Customer getACustomerAnyCustomer(Random random) {
+ return customersById.get(random.nextInt(customersById.size()));
+ }
+
+ /**
+ *
+ * Address address = alwaysGetAddress(street1, street2, city, state, zip,
+ * countryName);
+ * return createCustomer(fname, lname, address, phone, email,
+ * new Date(now), new Date(now), new Date(now),
+ * new Date(now + 7200000 ), discount, birthdate,
+ * data);
+ *
+ *
+ * Este método irá retornar o método de criação do controle do consumidor em
+ * relação a data de cadastro, último acesso, login e tempo de expiração da
+ * sessão.
+ *
+ * @param fname Primeiro nome do consumidor
+ * @param lname Sobrenome / Último nome do consumidor
+ * @param street1 Rua/Avenida do endereço
+ * @param street2 Rua/Avenida do endereço (complemento)
+ * @param city Cidade do endereço
+ * @param state Estado
+ * @param zip Código postal
+ * @param countryName Nome do País
+ * @param phone Telefone
+ * @param email E-mail
+ * @param discount Desconto
+ * @param birthdate Data de Nascimento
+ * @param data Dado referente ao Consumidor
+ * @param now Hora Atual
+ * @return O método createCustomer com os parâmetros: fname, lname, address,
+ * phone, email, since, lastVisit, login, expiration, discount, birthdate,
+ * data)
+ */
+ public static Customer createCustomer(String fname, String lname, String street1,
+ String street2, String city, String state, String zip,
+ String countryName, String phone, String email, double discount,
+ Date birthdate, String data, long now) {
+ Address address = alwaysGetAddress(street1, street2, city, state, zip,
+ countryName);
+ return createCustomer(fname, lname, address, phone, email,
+ new Date(now), new Date(now), new Date(now),
+ new Date(now + 7200000 /* 2 hours */), discount, birthdate,
+ data);
+ }
+
+ /**
+ * Create a customer and inser it on customer Id list and customer username
+ * map.
+ *
+ * int id = customersById.size();
+ * String uname = TPCW_Util.DigSyl(id, 0);
+ * Customer customer = new Customer(id, uname, uname.toLowerCase(), fname,
+ * lname, phone, email, since, lastVisit, login, expiration,
+ * discount, 0, 0, birthdate, data, address);
+ * customersById.add(customer);
+ * customersByUsername.put(uname, customer);
+ * return customer;
+ *
+ *
+ * @param fname Primeiro nome do Consumidor
+ * @param lname Sobrenome / Último nome do consumidor
+ * @param address Endereço do consumidor
+ * @param phone Telefone
+ * @param email Email
+ * @param since Data de Cadastro
+ * @param lastVisit Data do último acesso
+ * @param login Data de acesso ao login da sessão
+ * @param expiration Data de expiração da sessão
+ * @param discount Desconto
+ * @param birthdate Data de Nascimento
+ * @param data Dado referente ao Consumidor
+ * @return customer Consumidor
+ */
+ private static Customer createCustomer(String fname, String lname, Address address,
+ String phone, String email, Date since, Date lastVisit,
+ Date login, Date expiration, double discount, Date birthdate,
+ String data) {
+ int id = customersById.size();
+ String uname = TPCW_Util.DigSyl(id, 0);
+ Customer customer = new Customer(id, uname, uname.toLowerCase(), fname,
+ lname, phone, email, since, lastVisit, login, expiration,
+ discount, 0, 0, birthdate, data, address);
+ customersById.add(customer);
+ customersByUsername.put(uname, customer);
+ return customer;
+ }
+
+ /**
+ * Updates the customer login expiring date aka refresh session.
+ *
+ * Customer customer = getCustomer(cId);
+ * if (customer != null) {
+ * customer.setLogin(new Date(now));
+ * customer.setExpiration(new Date(now + 7200000 ));
+ * }
+ *
+ *
+ * @param cId Id do Consumidor
+ * @param now Tempo / hora atual
+ */
+ public static void refreshCustomerSession(int cId, long now) {
+ Customer customer = getCustomer(cId);
+ if (customer != null) {
+ customer.setLogin(new Date(now));
+ customer.setExpiration(new Date(now + 7200000 /* 2 hours */));
+ }
+ }
+
+ /**
+ * Este método retorna um autor de maneira randômica.
+ *
+ * @param random Dado randômico / aleatório
+ * @return authorsById Autor do Id escolhido
+ */
+ private static Author getAnAuthorAnyAuthor(Random random) {
+ return authorsById.get(random.nextInt(authorsById.size()));
+ }
+
+ /**
+ * Este método cria um autor através dos parâmetros: nome completo (primeiro
+ * nome, nome do meio, e último nome / sobrenome), data de nascimento, e
+ * biografia do autor.
+ *
+ * @param fname Primeiro nome do Autor
+ * @param mname Nome do meio do Autor
+ * @param lname Sobrenome / Último nome do Autor
+ * @param birthdate Data de Nascimento
+ * @param bio Biografia do Autor
+ * @return author Autor
+ */
+ private static Author createAuthor(String fname, String mname, String lname,
+ Date birthdate, String bio) {
+ Author author = new Author(fname, mname, lname, birthdate, bio);
+ authorsById.add(author);
+ return author;
+ }
+
+ /**
+ * Este método é utilizado para recuperar um livro com base em seu índice de
+ * inserção
+ *
+ * @param bId id que representa o índice do book no sistema
+ * @return retorna optional do livro correspondente ao índice
+ */
+ public static Optional getBook(int bId) {
+ return Optional.ofNullable(booksById.get(bId));
+ }
+
+ /**
+ * Returns a list of recommeded books based on Users
+ *
+ * @param c_id Customer id
+ * @return Não implementado, Null
+ */
+ public static List getRecommendationByItens(int c_id) {
+ return null;
+ }
+
+ /**
+ *
+ * @param c_id
+ * @return
+ */
+ public static List getRecommendationByUsers(int c_id) {
+ return null;
+ }
+
+ /**
+ * Randomly rturns a book based on a Random object as the random engine
+ *
+ * @param random - um valor random
+ * @return uma instância de Book
+ */
+ public static Book getABookAnyBook(Random random) {
+ return booksById.get(random.nextInt(booksById.size()));
+ }
+
+ /**
+ *
+ * ArrayList<Book> books = new ArrayList<>();
+ * for (Book book : booksById) {
+ * if (subject.equals(book.getSubject())) {
+ * books.add(book);
+ * if (books.size() > 50) {
+ * break;
+ * }
+ * }
+ * }
+ * Collections.sort(books, (Book a, Book b) -> a.getTitle().compareTo(b.getTitle()));
+ * return books;
+ *
+ *
+ * @param subject - o assunto do livro
+ * @return uma lista de livros
+ */
+ public static List getBooksBySubject(String subject) {
+ ArrayList books = new ArrayList<>();
+ for (Book book : booksById) {
+ if (subject.equals(book.getSubject())) {
+ books.add(book);
+ if (books.size() > 50) {
+ break;
+ }
+ }
+ }
+ Collections.sort(books, (Book a, Book b) -> a.getTitle().compareTo(b.getTitle()));
+ return books;
+ }
+
+ /**
+ * Returns a sorted list of Books which contains the title argument as its
+ * own title or a subpart of the title with a maximum size of 51 Books.
+ *
+ * @param title - o título do livro
+ * @return uma lista de livros
+ */
+ public static List getBooksByTitle(String title) {
+ Pattern regex = Pattern.compile("^" + title);
+ ArrayList books = new ArrayList<>();
+ for (Book book : booksById) {
+ if (regex.matcher(book.getTitle()).matches()) {
+ books.add(book);
+ if (books.size() > 50) {
+ break;
+ }
+ }
+ }
+ Collections.sort(books, (Book a, Book b) -> a.getTitle().compareTo(b.getTitle()));
+ return books;
+ }
+
+ /**
+ * Returns a list of books that were written by an specific author.
+ *
+ * @param author - o autor do livro
+ * @return uma lista de livros
+ */
+ public static List getBooksByAuthor(String author) {
+ Pattern regex = Pattern.compile("^" + author);
+ ArrayList books = new ArrayList<>();
+ for (Book book : booksById) {
+ if (regex.matcher(book.getAuthor().getLname()).matches()) {
+ books.add(book);
+ if (books.size() > 50) {
+ break;
+ }
+ }
+ }
+ Collections.sort(books, (Book a, Book b) -> a.getTitle().compareTo(b.getTitle()));
+ return books;
+ }
+
+ /**
+ * Retorna os 50 livros mais recentes (PubDate) por assunto
+ *
+ * @param subject - o assunto do livro
+ * @return uma lista de livros
+ */
+ public static List getNewBooks(String subject) {
+ ArrayList books = new ArrayList<>();
+ for (Book book : booksById) {
+ if (subject.equals(book.getSubject())) {
+ books.add(book);
+ }
+ }
+ Collections.sort(books, new Comparator() {
+ public int compare(Book a, Book b) {
+ int result = b.getPubDate().compareTo(a.getPubDate());
+ if (result == 0) {
+ result = a.getTitle().compareTo(b.getTitle());
+ }
+ return result;
+ }
+ });
+ return books.subList(0, books.size() >= 50 ? 50 : books.size());
+ }
+
+
+
+ /**
+ * Counter Object to help on counting books processes.
+ *
+ */
+ protected static class Counter {
+
+ /**
+ *
+ */
+ public Book book;
+
+ /**
+ *
+ */
+ public int count;
+ }
+
+ /**
+ * Para realizar a busca por bestSellers o sistema aproveita do
+ * relacionamento de {@linkplain OrderLine} com {@linkplain Book}. É
+ * assumido que cada OrderLine possui apenas 01 {@linkplain Book}, neste
+ * caso a quantidade de vendas é identificada por esta relação. Sempre que
+ * acontece a criação de uma orderLine, é assumido que uma compra foi feita.
+ *
+ * É utilizado um limite de 100 livros para o resultado da pesquisa para
+ * evitar desperdício de memória no retorno do método. Este limite é
+ * estipulado por
+ *
+ * Com isto, é importante salientar que este método utiliza as vendas desta
+ * instância para recuperar os livros vendidos.
+ *
+ * @param subject Assunto que será utilizado para filtro de pesquisa por
+ * melhores vendedores
+ * @return Retorna uma lista dos livros mais vendidos desta
+ * {@linkplain Bookstore} com tamanho limitado em 100
+ */
+ public List getBestSellers(String subject) {
+ return null;
+ }
+
+ /**
+ * Returns a list of Orders.
+ *
+ * @return a list of Orders
+ */
+ public List getOrdersById() {
+ return ordersById;
+ }
+
+ /**
+ *
+ * @param subject
+ * @return
+ */
+
+
+ private static Book createBook(String title, Date pubDate, String publisher,
+ String subject, String desc, String thumbnail,
+ String image, double srp, Date avail, String isbn,
+ int page, String backing, String dimensions, Author author) {
+ int id = booksById.size();
+ Book book = new Book(id, title, pubDate, publisher, subject, desc,
+ thumbnail, image, srp, avail, isbn, page, backing,
+ dimensions, author);
+ booksById.add(book);
+ return book;
+ }
+
+ /**
+ * Updates Book image path and Thumbnail path of a Book
+ *
+ * @param bId - Book Id
+ * @param image - Image path
+ * @param thumbnail - thumbnail
+ * @param now - Publication Date.
+ */
+ public static void updateBook(int bId, String image,
+ String thumbnail, long now) {
+ Book book = getBook(bId).get();
+ book.setImage(image);
+ book.setThumbnail(thumbnail);
+ book.setPubDate(new Date(now));
+ //updateRelatedBooks(book);
+ }
+
+ /**
+ * Upadtes the stock of a Book based on Book Id and updates its cost.
+ *
+ * @param bId - Book Id
+ * @param cost - o custo
+ */
+ public void updateStock(int bId, double cost) {
+ Book book = getBook(bId).get();
+ if (!stockByBook.containsKey(book)) {
+ int stock = TPCW_Util.getRandomInt(rand, 10, 30);
+ stockByBook.put(book, new Stock(this.id, book, cost, stock));
+ }
+ stockByBook.get(book).setCost(cost);
+ }
+
+ /**
+ * Returns the stock of a Book based on Book Id.
+ *
+ * @param bId - Book Id
+ * @return uma instância de Stock
+ */
+ public Stock getStock(int bId) {
+ final Book book = getBook(bId).get();
+ final Stock stock = stockByBook.get(book);
+ return stock;
+ }
+
+ /**
+ * For all the clients that bought this book in the last 10000 orders, what
+ * are the five most sold books except this one.
+ *
+ * @param targetBook - O livro que terá os relacionados atualizados
+ *
+ */
+ private void updateRelatedBooks(Book targetBook) {
+ HashSet clientIds = new HashSet<>();
+ int j = 0;
+ Iterator i = ordersByCreation.iterator();
+ while (i.hasNext() && j <= 10000) {
+ Order order = i.next();
+ for (OrderLine line : order.getLines()) {
+ Book book = line.getBook();
+ if (targetBook.getId() == book.getId()) {
+ clientIds.add(order.getCustomer().getId());
+ break;
+ }
+ }
+ j++;
+ }
+ HashMap counters = new HashMap<>();
+ i = ordersByCreation.iterator();
+ while (i.hasNext()) {
+ Order order = i.next();
+ if (clientIds.contains(order.getCustomer().getId())) {
+ order.getLines().forEach((line) -> {
+ Book book = line.getBook();
+ if (targetBook.getId() != book.getId()) {
+ Counter counter = counters.get(book.getId());
+ if (counter == null) {
+ counter = new Counter();
+ counter.book = book;
+ counter.count = 0;
+ counters.put(book.getId(), counter);
+ }
+ counter.count += line.getQty();
+ }
+ });
+ }
+ }
+ Counter[] sorted = counters.values().toArray(new Counter[]{});
+ Arrays.sort(sorted, (Counter a, Counter b) -> {
+ if (b.count > a.count) {
+ return 1;
+ }
+ return b.count < a.count ? -1 : 0;
+ });
+ Book[] related = new Book[]{targetBook, targetBook, targetBook,
+ targetBook, targetBook};
+ for (j = 0; j < 5 && j < sorted.length; j++) {
+ related[j] = sorted[j].book;
+ }
+ targetBook.setRelated1(related[0]);
+ targetBook.setRelated2(related[1]);
+ targetBook.setRelated3(related[2]);
+ targetBook.setRelated4(related[3]);
+ targetBook.setRelated5(related[4]);
+ }
+
+ /**
+ * Returns the Shopping cart.
+ *
+ * @param id - Cart Id.
+ * @return uma instância de Cart
+ */
+ public Cart getCart(int id) {
+ return cartsById.get(id);
+ }
+
+ /**
+ * Creates a Shopping cart
+ *
+ * @param now - Date from now.
+ * @return uma instância de Cart
+ */
+ public Cart createCart(long now) {
+ int idCart = cartsById.size();
+ Cart cart = new Cart(idCart, new Date(now));
+ cartsById.add(cart);
+ return cart;
+ }
+
+ /**
+ * Update shopping cart for one book or many books.
+ *
+ * @param cId - cart Id
+ * @param bId - book Id
+ * @param bIds - books Id
+ * @param quantities - quantities if many books.
+ * @param now - Date from now.
+ * @return uma instância de Cart
+ */
+ public Cart cartUpdate(int cId, Integer bId, List bIds,
+ List quantities, long now) {
+ Cart cart = getCart(cId);
+
+ if (bId != null) {
+ cart.increaseLine(stockByBook.get(getBook(bId).get()), getBook(bId).get(), 1);
+ }
+
+ for (int i = 0; i < bIds.size(); i++) {
+ cart.changeLine(stockByBook.get(getBook(bId).get()), booksById.get(bIds.get(i)), quantities.get(i));
+ }
+
+ cart.setTime(new Date(now));
+
+ return cart;
+ }
+
+ /**
+ * Este método irá finalizar a ordem do pedido de compra através dos
+ * parâmetros: consumidor, dados do carrinho de compras, do cartão de
+ * crédito e do envio do produto.
+ *
+ * O método irá finalizar o pedido, enviar a transferência do cartão de
+ * crédito e criar uma ordem para que o produto seja enviado.
+ *
+ * @param customerId ID do Consumidor
+ * @param cartId ID do Carrinho de Compras
+ * @param comment Comentário
+ * @param ccType Tipo de Cartão de Crédito
+ * @param ccNumber Número do Cartão de Crédito
+ * @param ccName Name do titular do Cartão de Crédito
+ * @param ccExpiry Data de Expiração do Cartão de Crédito
+ * @param shipping Remessa do Pedido
+ * @param shippingDate Data de Envio
+ * @param addressId Endereço do Consumidor
+ * @param now Tempo / hora atual
+ * @return O método createOrder com os parâmetros: Consumidor, Data atual,
+ * carrinho de compras, comentários, dados para envio da ordem, e a
+ * transação do cartão.
+ */
+ public Order confirmBuy(int customerId, int cartId, String comment,
+ String ccType, long ccNumber, String ccName, Date ccExpiry,
+ String shipping, Date shippingDate, int addressId, long now) {
+ Customer customer = getCustomer(customerId);
+ Cart cart = getCart(cartId);
+ Address shippingAddress = customer.getAddress();
+ if (addressId != -1) {
+ shippingAddress = addressById.get(addressId);
+ }
+ cart.getLines().stream().map((cartLine) -> {
+ Book book = cartLine.getBook();
+ stockByBook.get(book).addQty(-cartLine.getQty());
+ return book;
+ }).filter((book) -> (stockByBook.get(book).getQty() < 10)).forEachOrdered((book) -> {
+ stockByBook.get(book).addQty(21);
+ });
+ CCTransaction ccTransact = new CCTransaction(ccType, ccNumber, ccName,
+ ccExpiry, "", cart.total(customer.getDiscount()),
+ new Date(now), shippingAddress.getCountry());
+ return createOrder(customer, new Date(now), cart, comment, shipping,
+ shippingDate, "Pending", customer.getAddress(),
+ shippingAddress, ccTransact);
+ }
+
+ private Order createOrder(Customer customer, Date date, Cart cart,
+ String comment, String shipType, Date shipDate,
+ String status, Address billingAddress, Address shippingAddress,
+ CCTransaction cc) {
+ int idOrder = ordersById.size();
+ Order order = new Order(idOrder, customer, date, cart, comment, shipType,
+ shipDate, status, billingAddress, shippingAddress, cc);
+ ordersById.add(order);
+ ordersByCreation.addFirst(order);
+ customer.logOrder(order);
+ cart.clear();
+ return order;
+ }
+
+
+
+ private static Random rand;
+
+ /**
+ * Randomly populates Addresses, Customers, Authors and Books lists.
+ *
+ * @param seed - um valor random
+ * @param now - data e hora atual
+ * @param items - a quantidde de itens
+ * @param customers - a quantidade de clientes
+ * @param addresses - a quantidade de enderços
+ * @param authors - a quantidade de autores
+ * @return true always
+ */
+ public static boolean populate(long seed, long now, int items, int customers,
+ int addresses, int authors) {
+ if (populated) {
+ return false;
+ }
+ System.out.println("Beginning TPCW population.");
+ rand = new Random(seed);
+ populateCountries();
+ populateAddresses(addresses, rand);
+ populateCustomers(customers, rand, now);
+ populateAuthorTable(authors, rand);
+ populateBooks(items, rand);
+ populateEvaluation(rand);
+ populated = true;
+ System.out.println("Finished TPCW population.");
+ return true;
+ }
+
+ /**
+ * Este método irá popular inicialmente quais países, taxas de câmbio, e
+ * moedas correntes são possíveis de serem buscados e cadastrados junto ao
+ * consumidor.
+ */
+ private static void populateCountries() {
+ String[] countries = {"United States", "United Kingdom", "Canada",
+ "Germany", "France", "Japan", "Netherlands",
+ "Italy", "Switzerland", "Australia", "Algeria",
+ "Argentina", "Armenia", "Austria", "Azerbaijan",
+ "Bahamas", "Bahrain", "Bangla Desh", "Barbados",
+ "Belarus", "Belgium", "Bermuda", "Bolivia",
+ "Botswana", "Brazil", "Bulgaria", "Cayman Islands",
+ "Chad", "Chile", "China", "Christmas Island",
+ "Colombia", "Croatia", "Cuba", "Cyprus",
+ "Czech Republic", "Denmark", "Dominican Republic",
+ "Eastern Caribbean", "Ecuador", "Egypt",
+ "El Salvador", "Estonia", "Ethiopia",
+ "Falkland Island", "Faroe Island", "Fiji",
+ "Finland", "Gabon", "Gibraltar", "Greece", "Guam",
+ "Hong Kong", "Hungary", "Iceland", "India",
+ "Indonesia", "Iran", "Iraq", "Ireland", "Israel",
+ "Jamaica", "Jordan", "Kazakhstan", "Kuwait",
+ "Lebanon", "Luxembourg", "Malaysia", "Mexico",
+ "Mauritius", "New Zealand", "Norway", "Pakistan",
+ "Philippines", "Poland", "Portugal", "Romania",
+ "Russia", "Saudi Arabia", "Singapore", "Slovakia",
+ "South Africa", "South Korea", "Spain", "Sudan",
+ "Sweden", "Taiwan", "Thailand", "Trinidad",
+ "Turkey", "Venezuela", "Zambia"};
+
+ double[] exchanges = {1, .625461, 1.46712, 1.86125, 6.24238, 121.907,
+ 2.09715, 1842.64, 1.51645, 1.54208, 65.3851,
+ 0.998, 540.92, 13.0949, 3977, 1, .3757,
+ 48.65, 2, 248000, 38.3892, 1, 5.74, 4.7304,
+ 1.71, 1846, .8282, 627.1999, 494.2, 8.278,
+ 1.5391, 1677, 7.3044, 23, .543, 36.0127,
+ 7.0707, 15.8, 2.7, 9600, 3.33771, 8.7,
+ 14.9912, 7.7, .6255, 7.124, 1.9724, 5.65822,
+ 627.1999, .6255, 309.214, 1, 7.75473, 237.23,
+ 74.147, 42.75, 8100, 3000, .3083, .749481,
+ 4.12, 37.4, 0.708, 150, .3062, 1502, 38.3892,
+ 3.8, 9.6287, 25.245, 1.87539, 7.83101,
+ 52, 37.8501, 3.9525, 190.788, 15180.2,
+ 24.43, 3.7501, 1.72929, 43.9642, 6.25845,
+ 1190.15, 158.34, 5.282, 8.54477, 32.77, 37.1414,
+ 6.1764, 401500, 596, 2447.7};
+
+ String[] currencies = {"Dollars", "Pounds", "Dollars", "Deutsche Marks",
+ "Francs", "Yen", "Guilders", "Lira", "Francs",
+ "Dollars", "Dinars", "Pesos", "Dram",
+ "Schillings", "Manat", "Dollars", "Dinar", "Taka",
+ "Dollars", "Rouble", "Francs", "Dollars",
+ "Boliviano", "Pula", "Real", "Lev", "Dollars",
+ "Franc", "Pesos", "Yuan Renmimbi", "Dollars",
+ "Pesos", "Kuna", "Pesos", "Pounds", "Koruna",
+ "Kroner", "Pesos", "Dollars", "Sucre", "Pounds",
+ "Colon", "Kroon", "Birr", "Pound", "Krone",
+ "Dollars", "Markka", "Franc", "Pound", "Drachmas",
+ "Dollars", "Dollars", "Forint", "Krona", "Rupees",
+ "Rupiah", "Rial", "Dinar", "Punt", "Shekels",
+ "Dollars", "Dinar", "Tenge", "Dinar", "Pounds",
+ "Francs", "Ringgit", "Pesos", "Rupees", "Dollars",
+ "Kroner", "Rupees", "Pesos", "Zloty", "Escudo",
+ "Leu", "Rubles", "Riyal", "Dollars", "Koruna",
+ "Rand", "Won", "Pesetas", "Dinar", "Krona",
+ "Dollars", "Baht", "Dollars", "Lira", "Bolivar",
+ "Kwacha"};
+
+ System.out.print("Creating " + countries.length + " countries...");
+
+ for (int i = 0; i < countries.length; i++) {
+ createCountry(countries[i], currencies[i], exchanges[i]);
+ }
+
+ System.out.println(" Done");
+ }
+
+ private static void populateAddresses(int number, Random rand) {
+ System.out.print("Creating " + number + " addresses...");
+
+ for (int i = 0; i < number; i++) {
+ if (i % 10000 == 0) {
+ System.out.print(".");
+ }
+ Country country = getACountryAnyCountry(rand);
+ createAddress(
+ TPCW_Util.getRandomString(rand, 15, 40),
+ TPCW_Util.getRandomString(rand, 15, 40),
+ TPCW_Util.getRandomString(rand, 4, 30),
+ TPCW_Util.getRandomString(rand, 2, 20),
+ TPCW_Util.getRandomString(rand, 5, 10),
+ country);
+ }
+
+ System.out.println(" Done");
+ }
+
+ private static void populateCustomers(int number, Random rand, long now) {
+ System.out.print("Creating " + number + " customers...");
+
+ for (int i = 0; i < number; i++) {
+ if (i % 10000 == 0) {
+ System.out.print(".");
+ }
+ Address address = getAnAddressAnyAddress(rand);
+ long since = now - TPCW_Util.getRandomInt(rand, 1, 730) * 86400000 /* a day */;
+ long lastLogin = since + TPCW_Util.getRandomInt(rand, 0, 60) * 86400000 /* a day */;
+ createCustomer(
+ TPCW_Util.getRandomString(rand, 8, 15),
+ TPCW_Util.getRandomString(rand, 8, 15),
+ address,
+ TPCW_Util.getRandomString(rand, 9, 16),
+ TPCW_Util.getRandomString(rand, 2, 9) + "@"
+ + TPCW_Util.getRandomString(rand, 2, 9) + ".com",
+ new Date(since),
+ new Date(lastLogin),
+ new Date(now),
+ new Date(now + 7200000 /* 2 hours */),
+ rand.nextInt(51),
+ TPCW_Util.getRandomBirthdate(rand),
+ TPCW_Util.getRandomString(rand, 100, 500));
+ }
+
+ System.out.println(" Done");
+ }
+
+ private static void populateAuthorTable(int number, Random rand) {
+ System.out.print("Creating " + number + " authors...");
+
+ for (int i = 0; i < number; i++) {
+ if (i % 10000 == 0) {
+ System.out.print(".");
+ }
+ createAuthor(
+ TPCW_Util.getRandomString(rand, 3, 20),
+ TPCW_Util.getRandomString(rand, 1, 20),
+ TPCW_Util.getRandomLname(rand),
+ TPCW_Util.getRandomBirthdate(rand),
+ TPCW_Util.getRandomString(rand, 125, 500));
+ }
+
+ System.out.println(" Done");
+ }
+
+ /**
+ * Este método irá popular quais assuntos são possíveis de serem buscados
+ * pelo consumidor.
+ */
+ private static final String[] SUBJECTS = {"ARTS", "BIOGRAPHIES", "BUSINESS", "CHILDREN",
+ "COMPUTERS", "COOKING", "HEALTH", "HISTORY",
+ "HOME", "HUMOR", "LITERATURE", "MYSTERY",
+ "NON-FICTION", "PARENTING", "POLITICS",
+ "REFERENCE", "RELIGION", "ROMANCE",
+ "SELF-HELP", "SCIENCE-NATURE", "SCIENCE_FICTION",
+ "SPORTS", "YOUTH", "TRAVEL"};
+ private static final String[] BACKINGS = {"HARDBACK", "PAPERBACK", "USED", "AUDIO",
+ "LIMITED-EDITION"};
+
+ private static void populateBooks(int number, Random rand) {
+
+ System.out.print("Creating " + number + " books...");
+
+ for (int i = 0; i < number; i++) {
+ if (i % 10000 == 0) {
+ System.out.print(".");
+ }
+ Author author = getAnAuthorAnyAuthor(rand);
+ Date pubdate = TPCW_Util.getRandomPublishdate(rand);
+ double srp = TPCW_Util.getRandomInt(rand, 100, 99999) / 100.0;
+ String subject = SUBJECTS[rand.nextInt(SUBJECTS.length)];
+ String title = subject + " " + TPCW_Util.getRandomString(rand, 14, 60);
+ createBook(
+ title,
+ pubdate,
+ TPCW_Util.getRandomString(rand, 14, 60),
+ SUBJECTS[rand.nextInt(SUBJECTS.length)],
+ TPCW_Util.getRandomString(rand, 100, 500),
+ "img" + i % 100 + "/thumb_" + i + ".gif",
+ "img" + i % 100 + "/image_" + i + ".gif",
+ srp,
+ new Date(pubdate.getTime()
+ + TPCW_Util.getRandomInt(rand, 1, 30) * 86400000 /* a day */),
+ TPCW_Util.getRandomString(rand, 13, 13),
+ TPCW_Util.getRandomInt(rand, 20, 9999),
+ BACKINGS[rand.nextInt(BACKINGS.length)],
+ (TPCW_Util.getRandomInt(rand, 1, 9999) / 100.0) + "x"
+ + (TPCW_Util.getRandomInt(rand, 1, 9999) / 100.0) + "x"
+ + (TPCW_Util.getRandomInt(rand, 1, 9999) / 100.0),
+ author);
+ }
+
+ for (int i = 0; i < number; i++) {
+ Book book = booksById.get(i);
+ HashSet related = new HashSet<>();
+ while (related.size() < 5) {
+ Book relatedBook = getABookAnyBook(rand);
+ if (relatedBook.getId() != i) {
+ related.add(relatedBook);
+ }
+ }
+ Book[] relatedArray = new Book[]{booksById.get(TPCW_Util.getRandomInt(rand, 0, number - 1)),
+ booksById.get(TPCW_Util.getRandomInt(rand, 0, number - 1)),
+ booksById.get(TPCW_Util.getRandomInt(rand, 0, number - 1)),
+ booksById.get(TPCW_Util.getRandomInt(rand, 0, number - 1)),
+ booksById.get(TPCW_Util.getRandomInt(rand, 0, number - 1))};
+ relatedArray = related.toArray(relatedArray);
+ book.setRelated1(relatedArray[0]);
+ book.setRelated2(relatedArray[1]);
+ book.setRelated3(relatedArray[2]);
+ book.setRelated4(relatedArray[3]);
+ book.setRelated5(relatedArray[4]);
+ }
+
+ System.out.println(" Done");
+ }
+
+ void populateInstanceBookstore(int number, Random rand, long now) {
+ populateOrders(number, rand, now);
+ populateStocks(number, rand, now);
+
+ }
+
+ private void populateStocks(int number, Random rand, long now) {
+ System.out.print("Creating " + number + " stocks...");
+ for (int i = 0; i < number; i++) {
+ if (i % 10000 == 0) {
+ System.out.print(".");
+ }
+ int nBooks = TPCW_Util.getRandomInt(rand, 1, 5);
+ for (int j = 0; j < nBooks; j++) {
+ Book book = getABookAnyBook(rand);
+ if (!stockByBook.containsKey(book)) {
+ double cost = TPCW_Util.getRandomInt(rand, 50, 100) / 100.0;
+ int quantity = TPCW_Util.getRandomInt(rand, 300, 400);
+ stockByBook.put(book, new Stock(this.id, book, cost, quantity));
+ }
+ }
+ }
+ System.out.println(" Done");
+ }
+
+ private void populateOrders(int number, Random rand, long now) {
+ String[] credit_cards = {"VISA", "MASTERCARD", "DISCOVER",
+ "AMEX", "DINERS"};
+ String[] ship_types = {"AIR", "UPS", "FEDEX", "SHIP", "COURIER",
+ "MAIL"};
+ String[] status_types = {"PROCESSING", "SHIPPED", "PENDING",
+ "DENIED"};
+
+ System.out.print("Creating " + number + " orders...");
+
+ for (int i = 0; i < number; i++) {
+ if (i % 10000 == 0) {
+ System.out.print(".");
+ }
+ int nBooks = TPCW_Util.getRandomInt(rand, 1, 5);
+ Cart cart = new Cart(-1, null);
+ String comment = TPCW_Util.getRandomString(rand, 20, 100);
+ for (int j = 0; j < nBooks; j++) {
+ Book book = getABookAnyBook(rand);
+ int quantity = TPCW_Util.getRandomInt(rand, 1, 300);
+ if (!stockByBook.containsKey(book)) {
+ double cost = TPCW_Util.getRandomInt(rand, 50, 100) / 100.0;
+ int stock = TPCW_Util.getRandomInt(rand, 300, 400);
+ stockByBook.put(book, new Stock(this.id, book, cost, stock));
+ }
+ cart.changeLine(stockByBook.get(book), book, quantity);
+ }
+
+ Customer customer = getACustomerAnyCustomer(rand);
+ CCTransaction ccTransact = new CCTransaction(
+ credit_cards[rand.nextInt(credit_cards.length)],
+ TPCW_Util.getRandomLong(rand, 1000000000000000L, 9999999999999999L),
+ TPCW_Util.getRandomString(rand, 14, 30),
+ new Date(now + TPCW_Util.getRandomInt(rand, 10, 730) * 86400000 /* a day */),
+ TPCW_Util.getRandomString(rand, 15, 15),
+ cart.total(customer.getDiscount()),
+ new Date(now),
+ getACountryAnyCountry(rand));
+ long orderDate = now - TPCW_Util.getRandomInt(rand, 53, 60) * 86400000 /* a day */;
+ long shipDate = orderDate + TPCW_Util.getRandomInt(rand, 0, 7) * 86400000 /* a day */;
+ createOrder(
+ customer,
+ new Date(orderDate),
+ cart, comment,
+ ship_types[rand.nextInt(ship_types.length)],
+ new Date(shipDate),
+ status_types[rand.nextInt(status_types.length)],
+ getAnAddressAnyAddress(rand),
+ getAnAddressAnyAddress(rand),
+ ccTransact);
+ }
+
+ System.out.println(" Done");
+ }
+
+ private static void populateEvaluation(Random rand) {
+
+ System.out.print("Creating ");
+ // to do
+
+ }
+
+}
diff --git a/src/main/java/util/TPCW_Util.java b/src/main/java/util/TPCW_Util.java
new file mode 100644
index 0000000..5780431
--- /dev/null
+++ b/src/main/java/util/TPCW_Util.java
@@ -0,0 +1,230 @@
+package util;
+
+
+import java.util.*;
+
+/**
+ * *
+ */
+public class TPCW_Util {
+
+ private static final String[] arrayFnames = new String[]{
+ "Alice", "Miguel", "Sophia", "Arthur", "Helena", "Bernardo", "Valentina",
+ "Heitor", "Laura", "Davi", "Isabella", "Lorenzo", "Manuela", "Théo",
+ "Júlia", "Pedro", "Heloísa", "Gabriel", "Luiza", "Enzo", "Maria",
+ "Luiza", "Matheus", "Lorena", "Lucas", "Lívia", "Benjamin", "Giovanna",
+ "Nicolas", "Marial", "Eduarda", "Guilherme", "Beatriz", "Rafael", "Mariali",
+ "Clara", "Joaquim", "Cecília", "Samuel", "Eloá", "Enzo", "Gabriel", "Lara",
+ "João", "Miguelito", "Maria", "Júlia", "Henrique", "Isadora", "Gustavo",
+ "Mariana", "Murilo", "Emanuelly", "Pedrosa", "Henrique", "Anana", "Júlia",
+ "Pietro", "Anani", "Luiza", "Lucca", "Anane", "Clara", "Felipe", "Melissa",
+ "João", "Pedrono", "Yasmin", "Isaac", "Mariari", "Alicy", "Benício", "Isabelly",
+ "Daniel", "Lavínia", "Anthony", "Esther", "Leonardo", "Sarah", "Davi",
+ "Lucca", "Sandro", "Elisa", "Bryan", "Antonella", "Eduardo", "Rafaela",
+ "João", "Lucas", "Marianna", "Cecília", "Victor", "Liz", "João", "Marina",
+ "Cauã", "Nicole", "Antônio", "Maitê", "Vicente", "Isis", "Caleb", "Alícia",
+ "Gael", "Luna", "Bento", "Rebeca", "Caio", "Agatha", "Emanuel", "Letícia",
+ "Vinícius", "Maria-Flor", "João", "Guilherme", "Gabriela", "Davi", "Lucas",
+ "Anna", "Laura", "Noah", "Catarina", "João", "Gabriel", "Clara", "João",
+ "Victor", "Ania", "Beatriz", "Luiz", "Miguela", "Vitória", "Francisco",
+ "Olívia", "Kaique", "Marianne", "Fernanda", "Otávio", "Emilly", "Augusto",
+ "Mariano", "Valentina", "Levi", "Milena", "Yuri", "Marianni", "Helena", "Enrico",
+ "Bianca", "Thiago", "Larissa", "Ian", "Mirella", "Victor", "Hugo", "Marianno",
+ "Flor", "Thomas", "Allana", "Henry", "Ania", "Sofia", "Luiz", "Felipe", "Clarice",
+ "Ryan", "Pietra", "Artur", "Miguelly", "Mariaty", "Vitória", "Davi", "Luiz",
+ "Maya", "Nathan", "Laís", "Pedrone", "Lucas", "Ayla", "Davi", "Miguelle", "Anne",
+ "Lívia", "Raul", "Eduarda", "Pedroso", "Miguelli", "Mariah", "Luiz", "Henrique",
+ "Stella", "Luan", "Anai", "Erick", "Gabrielly", "Martin", "Sophie", "Bruno",
+ "Carolina", "Rodrigo", "Mariallia", "Laura", "Luiz", "Gustavo", "Mariati", "Heloísa",
+ "Art", "Gabriel", "Mariami", "Soffia", "Breno", "Fernanda", "Kauê", "Malu", "Enzo",
+ "Miguelo", "Analu", "Fernando", "Amanda", "Arthuro", "Henrique", "Aurora", "Luiz",
+ "Otávio", "Mariassol", "Isis", "Carlos", "Eduardo", "Louise", "Tomás", "Heloise",
+ "Lucas", "Gabriel", "Ana", "Vitória", "André", "Ana", "Cecília", "José", "Anae",
+ "Liz", "Yago", "Joana", "Danilo", "Luana", "Anthony", "Gabriel", "Antônia",
+ "Ruan", "Isabel", "Miguelitto", "Henrique", "Bruna", "Oliver"
+ };
+ private static final String[] arrayLnames = new String[]{
+ "Ferreira",
+ "Braga",
+ "da Silva",
+ "Della Coletta",
+ "Zampirolli",
+ "Fernandes",
+ "Alves",
+ "Costalonga",
+ "Botteon",
+ "Caliman",
+ "de Oliveira",
+ "Zanette",
+ "Salvador",
+ "Silva",
+ "Zandonadi",
+ "Pesca",
+ "Falqueto",
+ "Tosi",
+ "da Costa",
+ "de Souza",
+ "Gomes",
+ "Calmon",
+ "Pereira",
+ "Sossai detto Pegorer",
+ "de Almeida",
+ "de Jesus",
+ "Martins",
+ "Balarini",
+ "Rodrigues",
+ "Gonçalves",
+ "Pizzol",
+ "Moreira",
+ "Vieira",
+ "Venturim",
+ "Bazoni",
+ "Filete",
+ "Almeida",
+ "Oliveira",
+ "dos Santos",
+ "Falchetto",
+ "Barbosa",
+ "Breda",
+ "Scaramussa",
+ "de Barros",
+ "Marques"};
+
+ /**
+ *
+ * @param rand
+ * @return
+ */
+ public static String getRandomLname(Random rand) {
+ final int index = getRandomInt(rand, 0, arrayLnames.length -1);
+ return arrayLnames[index];
+ }
+
+ /**
+ *
+ * @param rand
+ * @param min
+ * @param max
+ * @return
+ */
+ public static String getRandomString(Random rand, int min, int max) {
+ StringBuilder newstring = new StringBuilder();
+ final char[] chars = {'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k',
+ 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v',
+ 'w', 'x', 'y', 'z', 'A', 'B', 'C', 'D', 'E', 'F', 'G',
+ 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R',
+ 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z', '!', '@', '#',
+ '$', '%', '^', '&', '*', '(', ')', '_', '-', '=', '+',
+ '{', '}', '[', ']', '|', ':', ';', ',', '.', '?', '/',
+ '~', ' '}; //79 characters
+ int strlen = getRandomInt(rand, min, max);
+ for (int i = 0; i < strlen; i++) {
+ newstring.append(chars[rand.nextInt(chars.length)]);
+ }
+ return newstring.toString();
+ }
+
+ /**
+ *
+ * @param rand
+ * @param lower
+ * @param upper
+ * @return
+ */
+ public static int getRandomInt(Random rand, int lower, int upper) {
+ return rand.nextInt(upper - lower + 1) + lower;
+ }
+
+ /**
+ *
+ * @param rand
+ * @param lower
+ * @param upper
+ * @return
+ */
+ public static long getRandomLong(Random rand, long lower, long upper) {
+ return (long) (rand.nextDouble() * (upper - lower + 1) + lower);
+ }
+
+ /**
+ *
+ * @param rand
+ * @return
+ */
+ public static Date getRandomBirthdate(Random rand) {
+ return new GregorianCalendar(
+ TPCW_Util.getRandomInt(rand, 1880, 2000),
+ TPCW_Util.getRandomInt(rand, 0, 11),
+ TPCW_Util.getRandomInt(rand, 1, 30)).getTime();
+ }
+
+ /**
+ *
+ * @param rand
+ * @return
+ */
+ public static Date getRandomPublishdate(Random rand) {
+ return new GregorianCalendar(
+ TPCW_Util.getRandomInt(rand, 1930, 2000),
+ TPCW_Util.getRandomInt(rand, 0, 11),
+ TPCW_Util.getRandomInt(rand, 1, 30)).getTime();
+ }
+
+ /**
+ *
+ * @param d
+ * @param n
+ * @return
+ */
+ public static String DigSyl(int d, int n) {
+ StringBuilder resultString = new StringBuilder();
+ String digits = Integer.toString(d);
+
+ if (n > digits.length()) {
+ int padding = n - digits.length();
+ for (int i = 0; i < padding; i++) {
+ resultString = resultString.append("BA");
+ }
+ }
+
+ for (int i = 0; i < digits.length(); i++) {
+ switch (digits.charAt(i)) {
+ case '0':
+ resultString = resultString.append("BA");
+ break;
+ case '1':
+ resultString = resultString.append("OG");
+ break;
+ case '2':
+ resultString = resultString.append("AL");
+ break;
+ case '3':
+ resultString = resultString.append("RI");
+ break;
+ case '4':
+ resultString = resultString.append("RE");
+ break;
+ case '5':
+ resultString = resultString.append("SE");
+ break;
+ case '6':
+ resultString = resultString.append("AT");
+ break;
+ case '7':
+ resultString = resultString.append("UL");
+ break;
+ case '8':
+ resultString = resultString.append("IN");
+ break;
+ case '9':
+ resultString = resultString.append("NG");
+ break;
+ default:
+ break;
+ }
+ }
+
+ return resultString.toString();
+ }
+
+}
diff --git a/src/test/java/servico/BookstoreTest.java b/src/test/java/servico/BookstoreTest.java
new file mode 100644
index 0000000..8ce09e9
--- /dev/null
+++ b/src/test/java/servico/BookstoreTest.java
@@ -0,0 +1,418 @@
+package servico;
+
+import dominio.Address;
+import dominio.Author;
+import dominio.Book;
+import dominio.Cart;
+import dominio.Customer;
+import dominio.Order;
+import java.util.Arrays;
+import java.util.Date;
+import java.util.List;
+import java.util.Random;
+import org.junit.After;
+import org.junit.AfterClass;
+import org.junit.Before;
+import org.junit.BeforeClass;
+import org.junit.Test;
+import static org.junit.Assert.*;
+
+/**
+ *
+ * @author INF329
+ */
+public class BookstoreTest {
+
+ public BookstoreTest() {
+ }
+
+ static Bookstore instance;
+
+ @BeforeClass
+ public static void setUpClass() {
+
+ long seed = 0;
+ long now = System.currentTimeMillis();
+ int items = 10000;
+ int customers = 1000;
+ int addresses = 1000;
+ int authors = 100;
+ int orders = 10000;
+ Random rand = new Random(seed);
+ Bookstore.populate(seed, now, items, customers, addresses, authors);
+ instance = new Bookstore(0);
+ instance.populateInstanceBookstore(orders, rand, now);
+ }
+
+ @AfterClass
+ public static void tearDownClass() {
+ }
+
+ @Before
+ public void setUp() {
+ }
+
+ @After
+ public void tearDown() {
+ }
+
+ /**
+ * Test of isPopulated method, of class Bookstore.
+ */
+ @Test
+ public void testIsPopulated() {
+ System.out.println("isPopulated");
+ boolean expResult = true;
+ boolean result = instance.isPopulated();
+ assertEquals(expResult, result);
+ }
+
+ /**
+ * Test of alwaysGetAddress method, of class Bookstore.
+ */
+ @Test
+ public void testAlwaysGetAddress() {
+ System.out.println("alwaysGetAddress");
+ String street1 = "";
+ String street2 = "";
+ String city = "";
+ String state = "";
+ String zip = "";
+ String countryName = "";
+ Address result = instance.alwaysGetAddress(street1, street2, city, state, zip, countryName);
+ Address expResult = new Address(0, street1, street2, city, state, zip, result.getCountry());
+ assertEquals(expResult, result);
+
+ }
+
+ /**
+ * Test of getCustomer method, of class Bookstore.
+ */
+ @Test
+ public void testGetCustomer_int() {
+ System.out.println("getCustomer");
+ int cId = 0;
+ Customer result = instance.getCustomer(cId);
+ assertEquals(cId, result.getId());
+ }
+
+ /**
+ * Test of getCustomer method, of class Bookstore.
+ */
+ @Test
+ public void testGetCustomer_String() {
+ System.out.println("getCustomer");
+ String username = instance.getCustomer(10).getUname();
+ Customer result = instance.getCustomer(username).get();
+ assertEquals(username, result.getUname());
+
+ }
+
+ /**
+ * Test of createCustomer method, of class Bookstore.
+ */
+ @Test
+ public void testCreateCustomer() {
+ System.out.println("createCustomer");
+ String fname = "";
+ String lname = "";
+ String street1 = "";
+ String street2 = "";
+ String city = "";
+ String state = "";
+ String zip = "";
+ String countryName = "";
+ String phone = "";
+ String email = "";
+ double discount = 0.0;
+ Date birthdate = null;
+ String data = "";
+ long now = 0L;
+
+ Customer result = instance.createCustomer(fname, lname, street1, street2, city, state, zip, countryName, phone, email, discount, birthdate, data, now);
+ int id = result.getId();
+ String uname = result.getUname();
+ Date since = result.getSince();
+ Date lastVisit = result.getLastVisit();
+ Date login = result.getLogin();
+ Date expiration = result.getExpiration();
+ Address address = result.getAddress();
+ Customer expResult = new Customer(id, uname, uname.toLowerCase(), fname,
+ lname, phone, email, since, lastVisit, login, expiration,
+ discount, 0, 0, birthdate, data, address);
+ assertEquals(expResult, result);
+
+ }
+
+ /**
+ * Test of refreshCustomerSession method, of class Bookstore.
+ */
+ @Test
+ public void testRefreshCustomerSession() {
+ System.out.println("refreshCustomerSession");
+ int cId = 0;
+ long now = 0L;
+ instance.refreshCustomerSession(cId, now);
+ }
+
+ /**
+ * Test of getBook method, of class Bookstore.
+ */
+ @Test
+ public void testGetBook() {
+ System.out.println("getBook");
+ int bId = 0;
+ Book result = instance.getBook(bId).get();
+ assertEquals(bId, result.getId());
+
+ }
+
+ /**
+ * Test of getBooksBySubject method, of class Bookstore.
+ */
+ @Test
+ public void testGetBooksBySubject() {
+ System.out.println("getBooksBySubject");
+ String subject = "ARTS";
+ List result = instance.getBooksBySubject(subject);
+ assertEquals(result.size(), result.stream().filter(book -> book.getSubject().equals(subject)).count());
+
+ }
+
+ /**
+ * Test of getBooksByTitle method, of class Bookstore.
+ */
+ @Test
+ public void testGetBooksByTitle() {
+ System.out.println("getBooksByTitle");
+ String title = instance.getBook(0).get().getTitle().substring(0, 4);
+ List result = instance.getBooksByTitle(title);
+ assertEquals(result.size(), result.stream().filter(book -> book.getTitle().startsWith(title)).count());
+ }
+
+ /**
+ * Test of getBooksByAuthor method, of class Bookstore.
+ */
+ @Test
+ public void testGetBooksByAuthor() {
+ System.out.println("getBooksByAuthor");
+ Author author = instance.getBook(0).get().getAuthor();
+ List result = instance.getBooksByAuthor(author.getLname());
+ assertEquals(result.size(), result.stream().filter(book -> book.getAuthor().getLname().equals(author.getLname())).count());
+
+ }
+
+ /**
+ * Test of getNewBooks method, of class Bookstore.
+ */
+ @Test
+ public void testGetNewBooks() {
+ System.out.println("getNewBooks");
+ String subject = instance.getBook(0).get().getSubject();
+ List result = instance.getNewBooks(subject);
+ assertEquals(result.size(),
+ result.stream().filter(book -> book.getSubject().equals(subject)).count());
+
+ }
+
+ /**
+ * Test of updateBook method, of class Bookstore.
+ */
+ @Test
+ public void testUpdateBook() {
+ System.out.println("updateBook");
+ int bId = 0;
+ double cost = 0.0;
+ String image = "";
+ String thumbnail = "";
+ long now = 0L;
+ Book book = instance.getBook(bId).get();
+ instance.updateBook(bId, image, thumbnail, now);
+ assertEquals(bId, book.getId());
+ //assertEquals(cost, book.getCost(), 0.0);
+ assertEquals(image, book.getImage());
+ assertEquals(thumbnail, book.getThumbnail());
+ }
+
+ /**
+ * Test of getCart method, of class Bookstore.
+ */
+ // @Test
+ public void testGetCart() {
+ System.out.println("getCart");
+ int id = 0;
+
+ Cart expResult = null;
+ Cart result = instance.getCart(id);
+ assertEquals(expResult, result);
+
+ }
+
+ /**
+ * Test of createCart method, of class Bookstore.
+ */
+ private void testCreateCart() {
+ System.out.println("createCart");
+ long now = 0L;
+
+ Cart expResult = null;
+ Cart result = instance.createCart(now);
+ assertEquals(expResult, result);
+
+ }
+
+ /**
+ * Test of cartUpdate method, of class Bookstore.
+ */
+ // @Test
+ public void testCartUpdate() {
+ System.out.println("cartUpdate");
+ testCreateCart();
+ int cId = 0;
+ Integer bId = 1;
+ List bIds = Arrays.asList(10, 20);
+ List quantities = Arrays.asList(10, 20);
+ long now = 0L;
+
+ Cart expResult = null;
+ Cart result = instance.cartUpdate(cId, bId, bIds, quantities, now);
+ assertEquals(expResult, result);
+
+ }
+
+ /**
+ * Test of confirmBuy method, of class Bookstore.
+ */
+ // @Test
+ public void testConfirmBuy() {
+ System.out.println("confirmBuy");
+ int customerId = 0;
+ int cartId = 0;
+ String comment = "";
+ String ccType = "";
+ long ccNumber = 0L;
+ String ccName = "";
+ Date ccExpiry = null;
+ String shipping = "";
+ Date shippingDate = null;
+ int addressId = 0;
+ long now = 0L;
+ Order expResult = null;
+ Order result = instance.confirmBuy(customerId, cartId, comment, ccType, ccNumber, ccName, ccExpiry, shipping, shippingDate, addressId, now);
+ assertEquals(expResult, result);
+ }
+
+
+
+
+
+
+
+
+
+
+
+
+ /**
+ * Test of getId method, of class Bookstore.
+ */
+ //@Test
+ public void testGetId() {
+ System.out.println("getId");
+ Bookstore instance = null;
+ int expResult = 0;
+ int result = instance.getId();
+ assertEquals(expResult, result);
+ // TODO review the generated test code and remove the default call to fail.
+ fail("The test case is a prototype.");
+ }
+
+ /**
+ * Test of getRecommendationByItens method, of class Bookstore.
+ */
+ //@Test
+ public void testGetRecommendationByItens() {
+ System.out.println("getRecommendationByItens");
+ int c_id = 0;
+ List expResult = null;
+ List result = Bookstore.getRecommendationByItens(c_id);
+ assertEquals(expResult, result);
+ // TODO review the generated test code and remove the default call to fail.
+ fail("The test case is a prototype.");
+ }
+
+ /**
+ * Test of getRecommendationByUsers method, of class Bookstore.
+ */
+ //@Test
+ public void testGetRecommendationByUsers() {
+ System.out.println("getRecommendationByUsers");
+ int c_id = 0;
+ List expResult = null;
+ List result = Bookstore.getRecommendationByUsers(c_id);
+ assertEquals(expResult, result);
+ // TODO review the generated test code and remove the default call to fail.
+ fail("The test case is a prototype.");
+ }
+
+ /**
+ * Test of getABookAnyBook method, of class Bookstore.
+ */
+ //@Test
+ public void testGetABookAnyBook() {
+ System.out.println("getABookAnyBook");
+ Random random = null;
+ Book expResult = null;
+ Book result = Bookstore.getABookAnyBook(random);
+ assertEquals(expResult, result);
+ // TODO review the generated test code and remove the default call to fail.
+ fail("The test case is a prototype.");
+ }
+
+ /**
+ * Test of getOrdersById method, of class Bookstore.
+ */
+ //@Test
+ public void testGetOrdersById() {
+ System.out.println("getOrdersById");
+ Bookstore instance = null;
+ List expResult = null;
+ List result = instance.getOrdersById();
+ assertEquals(expResult, result);
+ // TODO review the generated test code and remove the default call to fail.
+ fail("The test case is a prototype.");
+ }
+
+
+
+ /**
+ * Test of updateStock method, of class Bookstore.
+ */
+ //@Test
+ public void testUpdateStock() {
+ System.out.println("updateStock");
+ int bId = 0;
+ double cost = 0.0;
+ Bookstore instance = null;
+ instance.updateStock(bId, cost);
+ // TODO review the generated test code and remove the default call to fail.
+ fail("The test case is a prototype.");
+ }
+
+
+
+ /**
+ * Test of populateInstanceBookstore method, of class Bookstore.
+ */
+ //@Test
+ public void testPopulateInstanceBookstore() {
+ System.out.println("populateInstanceBookstore");
+ int number = 0;
+ Random rand = null;
+ long now = 0L;
+ Bookstore instance = null;
+ instance.populateInstanceBookstore(number, rand, now);
+ // TODO review the generated test code and remove the default call to fail.
+ fail("The test case is a prototype.");
+ }
+}
From 9257f0a3e821b09bb0a0be96e3bf172c3513e1f8 Mon Sep 17 00:00:00 2001
From: esoft45 - Vitor Gomes da Silva
Date: Sat, 15 Feb 2025 15:06:47 -0300
Subject: [PATCH 2/2] Add Wiki HTML to the project
---
wikiIndex.html | 118 +++++++++++++++++++++++++++++++++++++++++++++++++
1 file changed, 118 insertions(+)
create mode 100644 wikiIndex.html
diff --git a/wikiIndex.html b/wikiIndex.html
new file mode 100644
index 0000000..e1992bf
--- /dev/null
+++ b/wikiIndex.html
@@ -0,0 +1,118 @@
+Wiki Grupo 07 INF329 2024
INF329g07

+
+
+
+
+
+Membros:
+
+
+Google Meet (https://meet.google.com/vdt-aaiu-kdx)
+KabanFlow (https://kanbanflow.com/board/4einBgC)
GitHub (https://github.com/gcesario203/Bookmarket-grupo07)
Jenkins (https://inf300.ic.unicamp.br/INF329/job/BookMarketCore07/)
+
ISSUES
+Sempre criar o card no board antes de começar a desenvolver!
+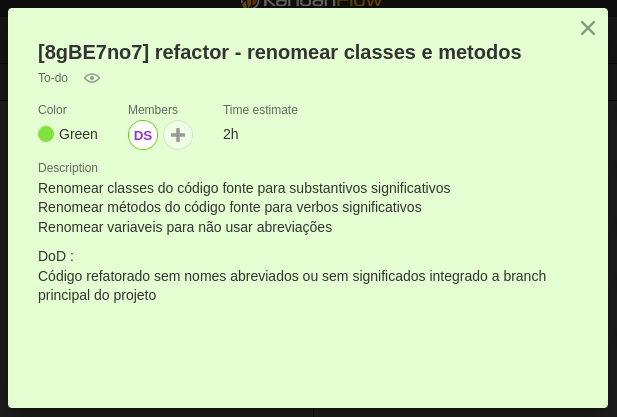
+
+Exemplo de template
+[Hash] Action - Summary
Description:
+Descrição da Task:
Subtasks (se houver):
+Definition of Done (DoD):
+Test instructions (se houver):
+Todo cartão precisa ter um responsável, se não for delegar para alguém quem criou é o responsável
+Campo de Resolution Description: Após feito deve ter o link para o PR e/ou outros links externos
+Em caso de feature é recomendado adicionar um campo de Test Instructions no cartão para que o revisor consiga testar a feature criada
+O hash pode ser obtido clicando em url e pegando a última parte do link
O cartão de exemplo foi criado para facilitar esse processo, podendo assim copiar ele e alterar os dados!
+Padrões de Cores
+DOC - Roxo 🟣
+FEAT - Verde 🟢
+REFACTOR - Amarelo 🟡
+REMOVE - Laranja 🟠
+SPIKE - Azul 🔵
+BUG - Vermelho 🔴
+
+WORFLOW
+
+Desenvolvimento
+
+
+ - Arrastar o card da coluna Do Sprint para In progress
+ - Dar pull na branch development
+ - Criar um branch cujo o nome seja o hash do cartão + nome legível da task que está trabalhando
+ - Fazer alterações e commitar ( o commit não deve conter muitas linhas e deverá conter uma mensagem descritiva do que foi realizado. Procure fazer vários commits. Não use git add .
+ - pense em quem vai revisar essa macarronada que vc vai subir)
+ - Ao concluir abra um PR para branch development
+ - Anexe o link do PR e outros links caso existam no campo Resolution Description do cartão
+ - Mova o cartão para coluna waiting review
+
+
+
+
+
+
+
+
+Revisão de atividades
+
+
+ - Mova o cartão para a coluna in Review
+ - Revise carinhosamente todo o código modificado
+ - Certifique-se de que o DoD está sendo cumprido
+ - Certifique-se de que não quebrou o código, teste as funções principais do sistema com asas alterações, ainda que não tenha feito alterações nelas diretamente
+ - Certifique-se de que o campo Resolution Description esteja preenchido
+ - Aceite o PR para branch development
+ - Acompanhe a execução dos testes na pipeline
+ - Em caso positivo, mova o cartão para Done, caso negativo, volte o cartão para Do sprint
+
+
+
+
+
+
+
+
+Clean Code e metodologia ágil sempre!
+Registro sobre as Sprints
+Um adendo sobre as Sprints do grupo 7 é que optamos por fazer em janelas de tempo menores, basicamente uma semana para cada.
+
+[SPRINT 1]
+
+
+ - Fizemos as primeiras adaptações da wiki no Moodle. Também fizemos uma revisão dos requisitos do projeto assíncrona com base no PDF. Por fim, montamos o Kanban com um card template.
+ - Criamos e estimamos nossos primeiros cards. Tomamos a decisão de como ficaria nossos repositórios locais e remotos, e também seus respectivos ambientes (dev, master, etc). Fizemos aprimoramentos na documentação da Wiki e primeiros cards relacionados ao código em si, que basicamente foram relacionados a refatoração, para melhor entendimento do time.
+
+[SPRINT 2]
+
+
+ - Ambiente de CI/CD no GitHub ficou completo, fizemos uma reavaliação dos requisitos do sistema por conta de dúvidas que surgiram e fizemos alguns testes que faltavam sua implementação.
+ - Melhoramento da Wiki adicionando informações das sprints e criações de métodos relacionados a BookStore e BookMarket, métodos de retorno de BestSellers, método de recomendação por itens e um teste de recomendação por usuário.
+ - Planning para quebra de cards dos métodos mais importantes de recomendação: getRecommendationByUser e ByItem.
+
+[SPRINT 3]
+
+
+ - Continuamos a fazer mais tasks de testes, relacionados ao BookStore e refatorações que serão importantes para fazer os métodos de recomendações.
+ - Também viemos trazer atualizações sobre as Sprints aqui na Wiki para manter atualizado sobre o que anda acontecendo durante cada semana.
+ - Implementação de GetBookPriceAverage e GetMinimumBookPrice para cálculo de preços de livros.
+ - Refatoração do método updateRelatedBooks no serviço BookStore.
+ - Adição de escopo de tipificação para Cliente.
+ - Implementação da ligação entre Customer, Cart e BookStore.
+
+[SPRINT 4]
+
+
+ - Fizemos a adição do GitInspector ao nosso projeto.
+ - Adição das imagens dos bagdes de tecnologias usadas que estão no GitHub aqui na Wiki.
+ - Implementação dos métodos getRecommendationByItens e getRecommendationByUsers
+
+
+
+
+
+
+
\ No newline at end of file